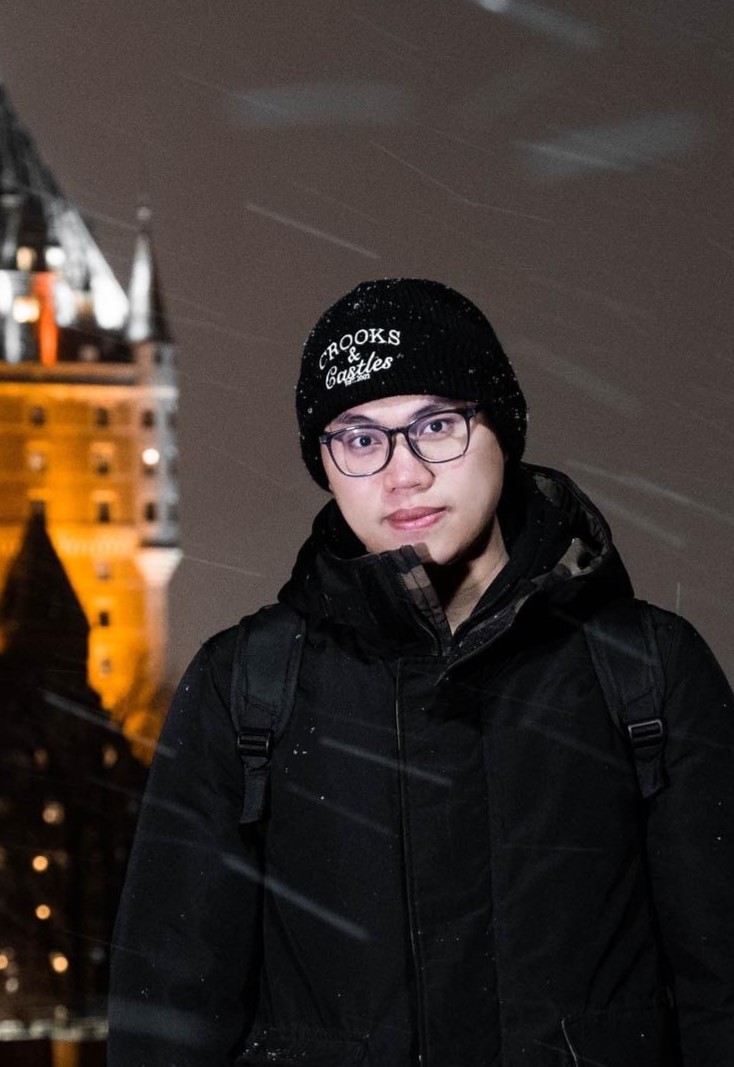
Chengyuan Zhang
Ph.D. candidate in the Department of Civil Engineering, McGill University
- Montreal, QC, Canada
- McGill University
- Google Scholar
- ORCID
- ResearchGate
- Github
You May Also Enjoy
Fundamental Probabilistic Graphical Models
less than 1 minute read
Published:
Tail-to-tail, head-to-tail, and head-to-head structures are three common types of graphical models. In this post, we will introduce these structures and discuss their implications for probabilistic modeling and inference.
Introduction to Autoregressive (AR) Processes
6 minute read
Published:
Autoregressive (AR) processes are a class of time series models used to describe a variable that is correlated with its past values. AR models are widely applied in various fields such as economics, engineering, finance, and traffic modeling, among others. In this post, we will introduce the concept of AR processes, their mathematical formulation, and the different types of AR models used in time series forecasting.
A Detailed Introduction to Gaussian Velocity Fields (GVF) Based on Gaussian Processes
7 minute read
Published:
In autonomous driving, modeling and understanding the interactions between the ego vehicle and its surrounding vehicles is crucial for safe and efficient navigation. One important challenge is dealing with varying traffic densities and dynamic environments, where the number of surrounding vehicles can fluctuate. Traditional models, which rely on a fixed number of surrounding vehicles, may struggle in such conditions.
Gaussian Processes (GP) for Time Series Forecasting
5 minute read
Published:
Time-series forecasting is a critical application of Gaussian Processes (GPs), as they offer a flexible and probabilistic framework for predicting future values in sequential data. GPs not only provide point predictions but also quantify uncertainty, making them particularly useful in scenarios where confidence in predictions is important.